How to Build Interactive Google Maps With R Shiny - A Complete Guide
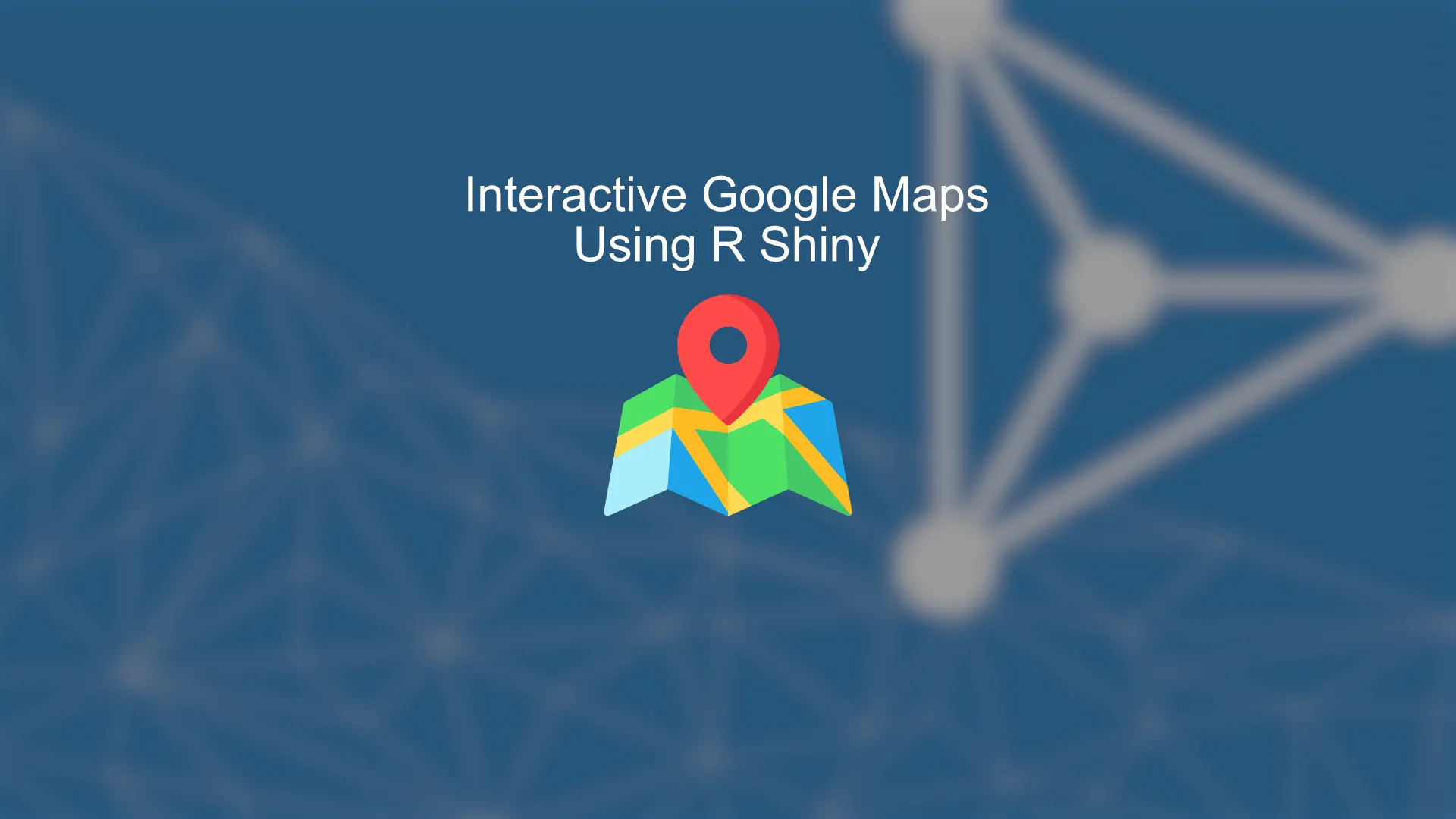
Visualizing spatial data in R is oftentimes more challenging than it initially seems. There’s a ton of moving parts, like map center point, zoom, map type, marker size, and so on. And then there's cartographic design and managing aesthetic appeal along with the functional elements. It goes without saying, but you have to get all of them right to have a cohesive map. That’s where this article comes in. We’ll show you how to build interactive Google Maps with R, and how to include them in R Shiny dashboards. With Google Maps in R, you can give your users a familiar map and retain customizability by placing it within a Shiny dashboard. To follow along, you’ll have to set up a Google Cloud account. It's fairly simple, but we'll show you how. <blockquote><strong>Looking for a Google Maps alternative? <a href="https://appsilon.com/leaflet-geomaps/" target="_blank" rel="noopener noreferrer">Check our guide on building interactive maps with R and Leaflet</a>.</strong></blockquote> Table of contents: <ul><li><a href="#google-cloud">How to Set up Google Cloud Account</a></li><li><a href="#maps-with-r">Build Interactive Google Maps with R</a></li><li><a href="#maps-with-r-shiny">Add Interactive Google Maps to R Shiny Dashboards</a></li><li><a href="#conclusion">Conclusion</a></li></ul> <hr /> <h2 id="google-cloud">How to Set up Google Cloud Account</h2> As the name suggests, Google Maps are developed by Google and offered as an API through their <a href="https://cloud.google.com/gcp/?utm_source=google&utm_medium=cpc&utm_campaign=emea-emea-all-en-dr-bkws-all-all-trial-e-gcp-1010042&utm_content=text-ad-none-any-DEV_c-CRE_500272531846-ADGP_Hybrid%20%7C%20BKWS%20-%20EXA%20%7C%20Txt%20~%20GCP%20~%20General%23v28-KWID_43700067001955397-kwd-454773864390-userloc_1007612&utm_term=KW_google%20map%20platform-NET_g-PLAC_&gclid=Cj0KCQiAys2MBhDOARIsAFf1D1e6keaUDzp724R0G63bcF4OvoMYz1V_osPKFU4fyzU5YxpbO9LIUcQaAsQuEALw_wcB&gclsrc=aw.ds" target="_blank" rel="noopener noreferrer">Cloud Platform</a>. You’ll have to register an account and set up billing. Yes, you do need to put in credit card information, but they won’t charge you a dime without your knowledge. Once you have an account and a project setup, you can enable APIs required for communication between R and Google. These are: <ul><li>Maps JavaScript API</li><li>Maps Static API</li><li>Geocoding API</li></ul> To enable them, click on the menu icon, go under <em>APIs & Services</em> and click on <em>Library</em>. You can search for the APIs from there and enable all three. Once done, you’ll see them listed on the main dashboard page: <img class="size-full wp-image-8809" src="https://webflow-prod-assets.s3.amazonaws.com/6525256482c9e9a06c7a9d3c%2F65b267129023e631a9959019_1-1.webp" alt="Image 1 - Enabled APIs on the Google Cloud Platform" width="858" height="352" /> Image 1 - Enabled APIs on the Google Cloud Platform Yours should show zeros under <em>Requests</em> if you’re setting up a new Google Cloud account. The final step is to create a new API key. Go under <em>Credentials</em> and click on <em>+ Create credentials</em> to get a new API key: <img class="size-full wp-image-8810" src="https://webflow-prod-assets.s3.amazonaws.com/6525256482c9e9a06c7a9d3c%2F65b2671239c9a87b7fcb724d_2-1.webp" alt="Image 2 - Creating Maps API key" width="3340" height="574" /> Image 2 - Creating Maps API key Once created, copy the string code somewhere safe. You’ll need it in the following section, where you’ll create a couple of interactive Google Maps with R. <h2 id="maps-with-r">Build Interactive Google Maps with R</h2> You’ll need the <code>googleway</code> library to follow along, so make sure to have it installed. Dataset-wise, you’ll use the <a href="https://www.kaggle.com/aravindram11/list-of-us-airports" target="_blank" rel="noopener noreferrer">US airports dataset from Kaggle</a>: <img class="size-full wp-image-8811" src="https://webflow-prod-assets.s3.amazonaws.com/6525256482c9e9a06c7a9d3c%2F65b7d4d9565df43d486a86af_715c1445_3-1.webp" alt="Image 3 - List of US Airports dataset" width="2814" height="1924" /> Image 3 - List of US Airports dataset Copy the following code snippet to load in the library and the data — don’t forget to change the working directory and the API key: <script src="https://gist.github.com/darioappsilon/bb6228b5b4db563c736c7f9a3c21f4e7.js"></script> Here are the first six dataset rows: <img class="size-full wp-image-8813" src="https://webflow-prod-assets.s3.amazonaws.com/6525256482c9e9a06c7a9d3c%2F65b2670b39c9a87b7fcb6e6b_1-2.webp" alt="Image 4 - Head of the US Airports dataset" width="1636" height="298" /> Image 4 - Head of the US Airports dataset The dataset has everything needed for map visualization. Latitude and longitude are generally everything you need, but you’ll see how the additional columns can make the map richer. <h3>Basic interactive Google Map with R</h3> Let’s start with the basics. We’ll add markers to every latitude and longitude combination from the dataset. You can use the <code>google_map()</code> function from <code>googleway</code> package to specify the API key and the dataset, and then call the <code>add_markers()</code> function to add the points: <script src="https://gist.github.com/darioappsilon/4101ce35b385a9fbd9cce8eae5c200df.js"></script> <img class="size-full wp-image-8814" src="https://webflow-prod-assets.s3.amazonaws.com/6525256482c9e9a06c7a9d3c%2F65b267158f849466f6bcf738_5-1.webp" alt="Image 5 - A basic map of US airport locations" width="2128" height="1566" /> Image 5 - A basic map of US airport locations There are many built-in options you don’t have to worry about. You can zoom in, zoom out, change the map type, and even use Google Street View! You can also tweak how the markers look like. We'll explore how, next. <h3>Change marker color</h3> The <code>googleway</code> library is quite restrictive with marker colors. You can go with red (default), blue, green, or lavender. To make matters worse, the package requires an additional column in the dataset for the color value. It’s useful if you’re using some conditional logic to color the markers, but a complete overkill if you aren’t. <script src="https://gist.github.com/darioappsilon/d7476a51fc701d3683d64cc3a0e834f4.js"></script> <img class="size-full wp-image-8815" src="https://webflow-prod-assets.s3.amazonaws.com/6525256482c9e9a06c7a9d3c%2F65b2671674660180239a65c5_6-1.webp" alt="Image 6 - Changing the marker color" width="2124" height="1570" /> Image 6 - Changing the marker color Long story short, you’re quite limited in color selection if you’re using <code>google</code> way to build interactive Google Maps with R. <h3>Add mouse over</h3> Adding mouse over effect to your interactive Google Maps with R is strongly advised if you want to make maps users will love. The mouse over effect represents what happens as the user pulls the mouse cursor over a marker. The code snippet below shows you how to add an airport name, stored in the <code>AIRPORT</code> column: <script src="https://gist.github.com/darioappsilon/fac0585fd66a2b96a041cac25fe00042.js"></script> <img class="size-full wp-image-8816" src="https://webflow-prod-assets.s3.amazonaws.com/6525256482c9e9a06c7a9d3c%2F65b267168533723b2a95a8b7_7.gif" alt="Image 7 - Adding mouse over text" width="1062" height="760" /> Image 7 - Adding mouse over text Mouse over effect can become a nightmare if your map has many markers, or if there’s almost no spacing between them. If that’s the case, you might want to consider info windows instead. <h3>Add info window</h3> Unlike mouse overs, info windows will activate only if the user clicks on a marker in your interactive map. Keep in mind — the info window also has to be closed manually by the user. To spice things up, we’ll add an additional column that contains the airport name, city, and state. You can add and customize yours easily with the <code>paste0()</code> function. <script src="https://gist.github.com/darioappsilon/f6362b91721b84f3ef1be3e1f72d7582.js"></script> <img class="size-full wp-image-8817" src="https://webflow-prod-assets.s3.amazonaws.com/6525256482c9e9a06c7a9d3c%2F65b267189609dcc98e633407_8.gif" alt="Image 8 - Adding info window on marker click" width="1062" height="760" /> Image 8 - Adding info window on marker click It’s hard to tell if info windows are better than mouse overs for this dataset, as markers aren’t packed extremely tightly. Go with the one that feels more natural. You now know the basics of interactive Google Maps with R and <code>googleway</code>. Next, you’ll see how to embed them to an R Shiny dashboard. <h2 id="maps-with-r-shiny">Add Interactive Google Maps to R Shiny Dashboards</h2> You’ll now see how easy it is to add interactive Google Maps to<a href="https://appsilon.com/shiny/" target="_blank" rel="noopener noreferrer"> R Shiny dashboards</a>. The one you’ll see below allows the user to specify a list of states from which the airports are displayed. Keep in mind: <ul><li><strong>App UI</strong> — You can use the <code>google_mapOutput()</code> function as a placeholder for the interactive Google map.</li><li><strong>App Server</strong> — Use the <code>renderGoogle_map()</code> function to display the map. The data is prepared earlier as a reactive component to match the user-selected states and display the airport, city, and state info on mouse over.</li></ul> Here’s the entire code: <script src="https://gist.github.com/darioappsilon/ee637f64fba7ea6b44415bad18042799.js"></script> <img class="size-full wp-image-8818" src="https://webflow-prod-assets.s3.amazonaws.com/6525256482c9e9a06c7a9d3c%2F65b01af34cedf11e5f959540_9.gif" alt="Image 9 - US Airports R Shiny dashboard" width="940" height="736" /> Image 9 - US Airports R Shiny dashboard The <code>googleway</code> library configures the location and the zoom level automatically for you. The map was zoomed to New York by default and changed instantly when we added more states. Overall, we have a decent-looking map for 30-something lines of code. <blockquote><strong>Learn how to use R to analyze large geolocation databases and build a <a href="https://appsilon.com/covid-19-risk-heat-maps-with-location-data-apache-arrow-markov-chain-modeling-and-r-shiny/" target="_blank" rel="noopener noreferrer">Corona Risk heat map - CoronaRank</a>.</strong></blockquote> The questions left to address are the speed and the looks. Luckily, we have dedicated articles on these topics, written with having R Shiny dashboards in mind: <ul><li><a href="https://appsilon.com/ux-design-of-shiny-apps-7-steps-to-design-dashboards-people-love/" target="_blank" rel="noopener noreferrer">UX Design for Shiny Apps</a></li><li><a href="https://appsilon.com/apache-arrow-in-r-supercharge-r-shiny-dashboards/" target="_blank" rel="noopener noreferrer">Apache Arrow in R for Supercharging Shiny Dashboards</a></li></ul> These two are excellent reads if you’ve enjoyed this article, as they allow you to take your R Shiny dashboards to the next level. <hr /> <h2 id="conclusion">Conclusion</h2> And there you have it — How to build, tweak, and style interactive Google Maps with R. You’ve learned a lot today, but there’s always room to improve. Here are a couple of challenges you can do next: <ul><li>Use custom icons instead of the default markers</li><li>Show airports as a heat map instead of markers</li><li>Calculate distances between airports using the Google Maps API</li></ul> Reference the <a href="https://rdrr.io/cran/googleway/" target="_blank" rel="noopener noreferrer">official documentation</a> if you decide to work on these challenges. And feel free to share your results with us - <a href="https://twitter.com/appsilon" target="_blank" rel="noopener noreferrer">@appsilon</a>. We’d love to see what you come up with. If you're looking for a faster way to showcase your map online, check out our <a href="https://templates.appsilon.com/" target="_blank" rel="noopener noreferrer">Shiny Dashboard Templates</a>. They're free to use and a great launching point for your project.
Contact us!
